How to use custom hook points with Codeigniter 3
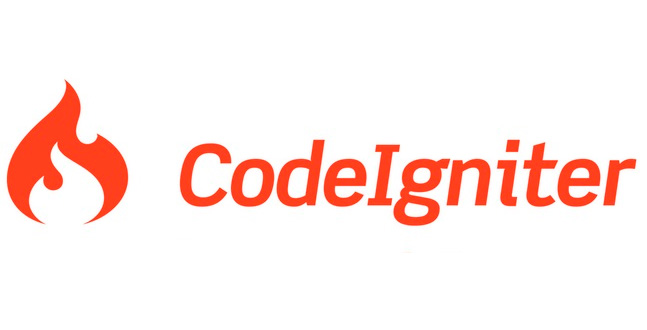
In this article, I will explain the process of implementing a custom hook point infrastructure allowing you to place custom hook points throughout functions in your controllers. This comes in handy when you want people to be able to modify a CodeIgniter 3 application without modifying the original controller files. The result is similar to the way WordPress lets developers modify it without changing the core files.
CodeIgniter comes with a built-in hook structure, however, the available hook points are defined by the framework and it’s not possible to add your own hook points. By default, there are only 7 hook points available (https://www.codeigniter.com/user_guide3/general/hooks.html).
What we want though, is the ability to add hook points wherever we’d like. Since this functionality is not supplied by CodeIgniter 3, I had to make a few tweaks.
The first steps are virtually the same compared to implementing regular CodeIgniter hooks.
STEP 1: MAKE SURE HOOKS ARE ENABLED
For hooks to be functional, we have to instruct CodeIgniter to enable the hook functionality. This is done by editing the main configuration file (typically located in /application/config/config.php) and set $config[‘enable_hooks’] to TRUE instead of FALSE.
STEP 2: CONFIGURE INDIVIDUAL HOOKS
Next, we’ll need to configure our individual hooks. CodeIgniter has a separate configuration file to declare our hooks in /application/config/hooks.php.
For each hook you want to configure, you will need to create an entry like the one below:
$hook[‘my_custom_hookpoint’][] = array(
'class' => 'MY_Hooks',
'function' => MyMethod,
'filename' => 'MY_Hooks.php',
'filepath' => 'hooks',
'params' => ''
);
The “my_custom_hookpoint” bit in $hook[‘my_custom_hookpoint’] connects this specific hook to a certain hook point; in this case, the hook point is a custom hook point named “my_custom_hookpoint”. We will explain how to declare your very own hook points later in this article.
The other items in this array are explained as follows:
‘CLASS’ => ‘MY_HOOKS’
The class item points to the class containing the function which will be called and executed at the hookpoint.
‘FUNCTION’ => ‘MYMETHOD’
The function item points to the function inside the specific class which is called and executed at the hook point.
‘FILENAME’ => ‘MY_HOOKS.PHP’
The filename item points to the file containing the class and function specified previously.
‘FILEPATH’ => ‘HOOKS’
The filepath item points to the path where your hook file is located.
‘PARAMS’ => ”
The params item can contain optional parameters passed to the hook function.
STEP 3: CREATE YOUR HOOK CODE
In this step, we’ll create the code which is executed when CodeIgniter runs into the specified hook point. Following the example from Step 2, we’ll be adding a file named “MY_Hooks.php” in the /application/hooks folder.
Inside that file, we’ll declare a class named “MY_Hooks” and within that class, we’ll add a function named “MyMethod”:
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class MY_Hooks {
public function MyMethod ()
{
echo "Hook works!";
}
}
So far, so good. The only missing piece of the puzzle is our custom hook point. As you can see in Step 1, we’re utilizing a hook point named “my_custom_hookpoint”. However, this is not a hook point provided by CodeIgniter. So if we were to stop here, our hook would not work.
What is left is figuring out how we can get CodeIgniter to allow us to specify our own custom hook points.
That last step is actually extremely simple! You start off by making sure you’re loading CodeIgniter’s core Hook class inside the controller in which you want to declare a custom hook point. You can do this either by loading the Hook class in the controller’s “__construct()” function or by loading it directly inside the function in which you want to declare your custom hook point.
My suggestion would be to load the Hook class in the “__construct()” function, as it will be available automatically in every function within that controller. However, the second method works fine as well.
To load the Hook class, use the following code:
$this->hooks =& load_class('Hooks', 'core');
Now that you have the Hook class available, all that’s left is to declare your custom hook point where you need it to be, by using the following code:
$this->hooks->call_hook('my_custom_hookpoint');
It’s important to realize that your hook code will get called on the exact same spot where you insert the above code.
ACCESSING THE CODEIGNITER FRAMEWORK INSIDE YOUR HOOK CODE
So far we have discussed how to configure hooks, setup your hook code, and add your very own hook points. However, the things we can achieve within our custom hook code are pretty limited at this point.
By default, the CodeIgniter framework is not available in our hooked function, which prevents us from accessing CodeIgniter’s database library and other essential parts of the framework.
To be able to access all the goodies CodeIgniter has to offer inside your hooked function, we will modify the class containing your hooked function as follows:
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class MY_Hooks {
private $CI;
public function __construct()
{
$this->CI =& get_instance();
}
public function MyMethod ()
{
echo "Hook works!";
}
}
Adding the __construc() function and loading a CodeIgniter instance and assigning it to “$this->CI” make the entire CodeIgniter framework available though “$this->Ci”.
For example, after modifying your hooks class as shown above, you would be able to access CodeIgniter’s configuration settings as follows:
echo $this->CI->config('base_url');
The above code would echo your application’s base URL to the browser.
Well, there you have it! A pretty nice and solid framework to add custom hook points to your application and therefore making modifications without messing with core application files a lot easier! We have been using this method in Pagestead for several weeks now and it’s been working great!